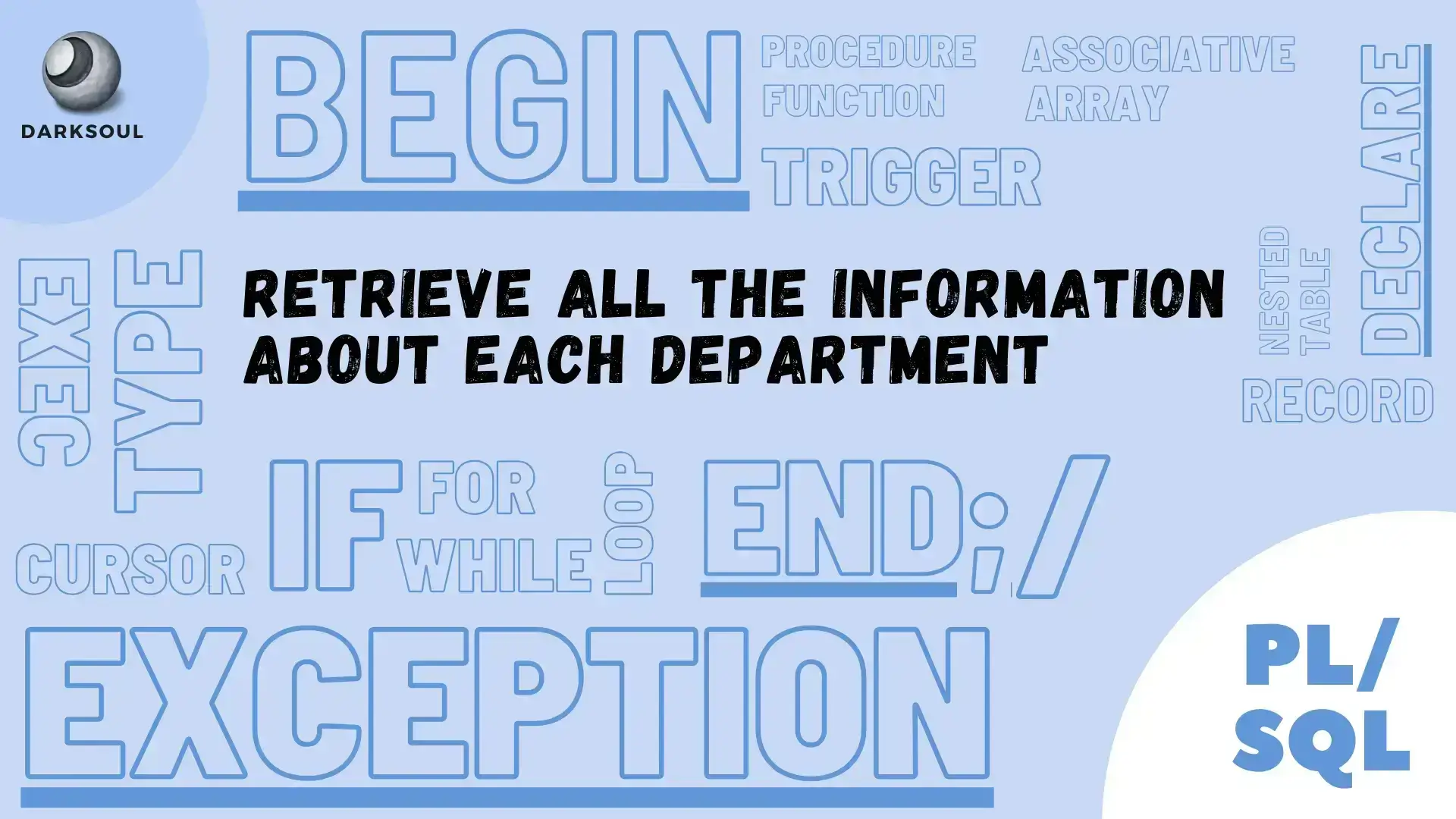
Create a PL/SQL block to retrieve all the information about each department from the DEPARTMENT table and display the information on the screen, incorporating a PL/SQL table of records.
COLUMN | DATATYPE | CONS. |
---|---|---|
DEPARTMENT_ID | NUMBER(5) | PK |
DEPARTMENT_NAME | VARCHAR2(25) | NOT NULL |
LOCATION_ID | VARCHAR2(15) |
Sample Output:
Department Details are : 1000, ADMIN, HQ-101 1010, DEVELOPMENT, CBE-103 10 DEVELOPMENT 1020, TESTING, CHN-102(Hint: Data is case sensitive. Use '/' to terminate the PL/SQL block)
Let's break down what's happening in this PL/SQL code step by step:
Setting up: The line SET SERVEROUTPUT ON; is like turning on a printer so that anything we ask the program to "print out" will actually show up.
Declaring variables: Here, we're creating three variables: depid, depname, and locid, which will hold the values of department ID, department name, and location ID respectively. These variables are based on the data types of these columns in the DEPARTMENT table.
Defining a cursor: Think of a cursor as a pointer or a tool that helps us go through the rows of a table one by one. Here, we're creating a cursor named dept that selects all columns from the DEPARTMENT table.
Starting the program: The BEGIN keyword marks the beginning of our actual PL/SQL program.
Printing a message: We're using DBMS_OUTPUT.PUT_LINE to print out the message "Department Details are :" to let the user know what kind of information they're about to see.
Opening the cursor: OPEN dept; is like opening a door to start using our cursor. Now we can start fetching rows from the DEPARTMENT table.
Looping through the rows: With LOOP, we're starting a loop that will continue until there are no more rows left in our cursor.
Fetching a row: FETCH dept INTO depid, depname, locid; grabs the values of depid, depname, and locid from the current row that the cursor is pointing to and stores them in our variables.
Checking for the end: EXIT WHEN dept%NOTFOUND; checks if we've reached the end of our cursor. If there are no more rows to fetch, the loop will stop.
Printing the row: DBMS_OUTPUT.PUT_LINE(depid || ', ' || depname || ', ' ||locid); prints out the values we fetched from the current row, separated by commas.
Ending the loop and program: Once we've gone through all the rows, the loop ends, and then the program ends with the /.
In essence, this code fetches information about departments from a table one row at a time and prints out each row's details.
Leave a comment